Tech
JsonObject.toString: Simplifying JSON Serialization in Java
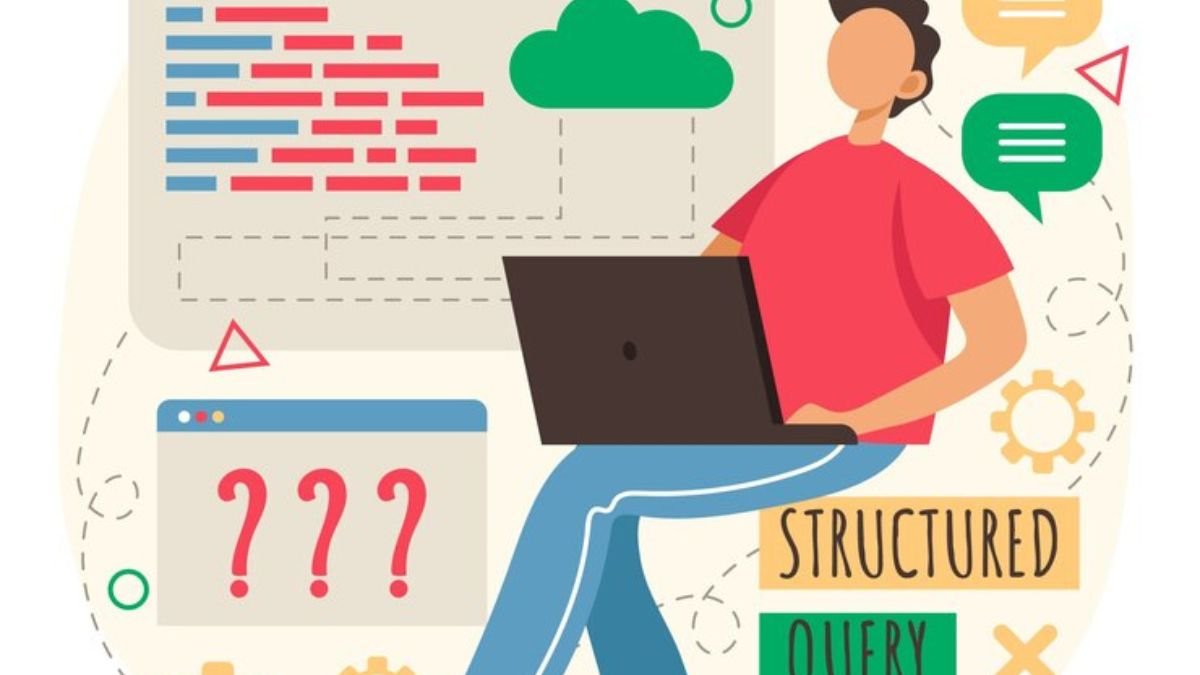
JSON (JavaScript Object Notation) has become a ubiquitous data interchange format, especially in web development. In Java, handling JSON data is made easier with the JsonObject class. One of the key methods in JsonObject is toString, which converts the object into its string representation. In this article, we’ll delve into the details of JsonObject.toString, its importance, usage, best practices, and more.
JsonObject is a class that the Java API for JSON processing (javax.json) provides to represent an immutable JSON object. It allows developers to work with JSON data in a structured manner, similar to how objects are manipulated in Java. The toString method in JsonObject is instrumental in converting the JSON object into its string equivalent.
Understanding JsonObject in Java
JsonObject encapsulates a JSON object, comprising key-value pairs where keys are strings and values can be various JSON types such as strings, numbers, arrays, or nested objects. It provides methods for accessing and manipulating these key-value pairs, making it a powerful tool for JSON handling in Java applications.
Importance of JsonObject.toString method
The toString method in JsonObject plays a vital role in serialization, converting the JSON object into a string that can be transmitted over networks or stored in files. It simplifies the process of generating JSON output, making it easier to work with JSON data in Java applications.
How to use JsonObject.toString effectively
To use JsonObject.toString effectively, developers should adhere to best practices such as properly formatting the JSON output, handling null values, and ensuring consistency in the output format. By following these guidelines, developers can create clean and readable JSON strings that are easy to parse and understand.
Best practices for using JsonObject.toString
When using JsonObject.toString, it’s essential to maintain consistency in the output format to ensure compatibility with other systems. Additionally, developers should consider performance implications, especially when dealing with large datasets, and optimize the serialization process accordingly.
Examples of JsonObject.toString in action
Let’s consider a simple example where we have a JsonObject representing a user profile:
javaCopy code
JsonObject profile = Json.createObjectBuilder() .add(“name”, “John Doe”) .add(“age”, 30) .add(“email”, “john@example.com”) .build(); String json = profile.toString(); System.out.println(json);
This code snippet creates a JsonObject with three key-value pairs and then converts it into a string using the toString method.
Advantages of using JsonObject.toString
Using JsonObject.toString offers several advantages, including simplicity, readability, and compatibility. It abstracts away the complexity of JSON serialization, allowing developers to focus on their application logic rather than low-level details of data formatting.
Potential pitfalls to avoid
While JsonObject.toString simplifies JSON serialization, developers should be cautious of potential pitfalls such as performance bottlenecks and security vulnerabilities. It’s essential to profile the application and optimize the serialization process to ensure optimal performance, especially in high-traffic systems.
JsonObject.toString vs. other similar methods
There are other methods for JSON serialization in Java, such as ObjectMapper in Jackson library or Gson in Google Gson library. While these libraries offer more features and flexibility, JsonObject.toString is lightweight and suitable for simple JSON serialization tasks.
Performance considerations
When using JsonObject.toString, developers should consider performance implications, especially when dealing with large datasets. Serializing complex objects can be resource-intensive, so optimizing the serialization process is crucial for maintaining application performance.
Security implications
Security is paramount when serializing JSON data, as it can expose sensitive information if not handled properly. Developers should sanitize input data to prevent injection attacks and ensure that only trusted sources can access the serialization functionality.
Compatibility with different JSON libraries
JsonObject.toString is compatible with various JSON libraries, making it easy to integrate into existing projects. However, developers should verify compatibility and ensure that the chosen library meets their project requirements in terms of performance, features, and community support.
JsonObject.toString in real-world applications
In real-world applications, JsonObject.toString is used extensively for serializing JSON data for transmission over networks, storing configuration settings, and persisting application state. Its simplicity and ease of use make it a popular choice among Java developers for handling JSON data.
Conclusion
JsonObject.toString is a valuable tool for simplifying JSON serialization in Java applications. By converting JSON objects into their string representations, it facilitates data interchange and storage, making it easier for developers to work with JSON data in their applications.
FAQs
What is the JsonObject class in Java?
JsonObject is a class that the Java API for JSON processing (javax.json) provides to represent an immutable JSON object.
How does the toString method work in JsonObject?
The toString method in JsonObject converts the JSON object into its string representation.
Can JsonObject.toString handle nested JSON objects?
Yes, JsonObject.toString can handle nested JSON objects by recursively serializing them into strings.
Is JsonObject.toString suitable for large datasets?
JsonObject.toString is suitable for small to moderate-sized datasets, but developers should optimize the serialization process for large datasets to avoid performance issues.
Are there any alternatives to JsonObject.toString for JSON serialization?
Yes, alternatives to JsonObject.toString include ObjectMapper in Jackson library and Gson in Google Gson library, which offer more features and flexibility for JSON serialization in Java.